Mastering the Basics: Arrays from the Ground Up

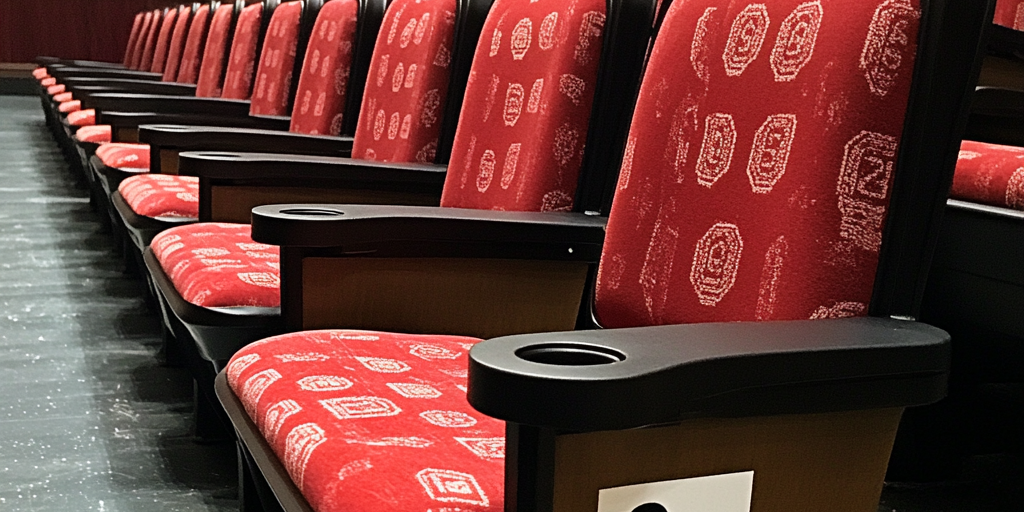

Understanding Data Structures Through Big O Notation
In our previous discussion on Big O notation, we explored how it helps us measure the efficiency of algorithms. Now, let’s take the next logical step: understanding data structures in the context of Big O.
Why Does Big O Matter for Data Structures?
Every data structure is built for specific tasks. Some are great at fast lookups, while others make inserting or removing items easy. But how do we know which one is best for the job? Big O notation helps us compare different data structures based on their efficiency.
For example:
- Need fast lookups? Arrays are a great choice.
- Need to frequently insert or remove items? Maybe another structure, like a linked list, is better.
By understanding how fast or slow different operations are, we can choose the right tool for the job.
What is an Array?
Imagine you’re sitting in a movie theater, and every seat has a number. If you have a ticket for Seat #5, you can go straight to it without checking every seat. That’s how arrays work!
An array is a list of values stored in a row, one after the other, in the computer’s memory. Since each value is in a fixed position, you can jump directly to any item if you know its index (just like a seat number).
Why Use Arrays?
Arrays are fast for certain tasks and slow for others. Let's break it down.
✅ Good for:
- Fast Access by Index → You can grab any item in an array instantly. (Big O: O(1))
- Easy to Loop Through → Arrays are stored in a straight line, making them great for loops.
- Foundation for Other Data Structures → Many advanced structures (like hash tables and queues) use arrays behind the scenes.
❌ Not good for:
- Frequent Insertions/Deletions in the Middle → If you insert a seat in the middle of a packed row, you have to move everyone down. This is slow! (Big O: O(n)O(n)O(n))
- Constantly Changing Size → In some languages, arrays have fixed sizes, making them tricky when the number of items changes often.
Time Complexity Overview
(Think of this as "How long does it take?" when working with an array.)
Operation | Big O Notation | Explanation |
---|---|---|
Access (indexing) | O(1) | Like knowing your seat number and going straight to it. |
Search (linear) | O(n) | Like scanning every seat to find your friend |
Binary Search (sorted arrays only) | O(log n) | Like dividing the theater in half each time until you find your friend. |
Insert/Delete (middle) | O(n) | Like inserting a new seat in the middle and shifting everyone. |
Example: Fast Searching with Binary Search
If the seats (array items) are sorted, we can use a smart trick called Binary Search to find a value way faster than scanning every seat.
Binary Search Code Example
1function binarySearch(arr: number[], target: number): number {
2 let left = 0;
3 let right = arr.length - 1;
4
5 while (left <= right) {
6 const mid = Math.floor((left + right) / 2);
7
8 if (arr[mid] === target) {
9 return mid; // Found the target seat
10 } else if (arr[mid] < target) {
11 left = mid + 1; // Look in the higher-numbered seats
12 } else {
13 right = mid - 1; // Look in the lower-numbered seats
14 }
15 }
16
17 return -1; // Seat not found
18}
19
20// Example usage:
21const seats = [1, 3, 5, 7, 9, 11];
22const targetSeat = 7;
23const foundIndex = binarySearch(seats, targetSeat);
24console.log(foundIndex); // Output: 3
Here’s how it works:
- Check the middle seat → Is it the one you need?
- If not, decide whether your seat is in the left half or right half.
- Ignore the half that doesn’t matter and repeat.
This way, we eliminate half of the possibilities each step—making search much faster.
🔹 Big O: O(log n) (Logarithmic Time) → It’s way better than searching one-by-one!
Common Mistakes (Pitfalls)
🚨 Mistake #1: Out-of-Bounds Errors
Trying to access array[10]
when there are only 5 items will cause an error.
🚨 Mistake #2: Slow Insertions/Deletions in the Middle
If you insert/remove an item in the middle, all the other seats shift—this takes time!
🚨 Mistake #3: Forgetting Sorting for Binary Search
Binary search only works if the array is sorted. If not, it won’t find the correct item.
Best Practices
✔ Use Arrays for Fast Access
- If you know the exact index of an item, an array is the best choice.
✔ Sort Your Array Before Searching
- If you plan to use binary search, make sure your array is sorted.
✔ Consider Other Structures for Frequent Insertions
- If you often add or remove items in the middle, a linked list might be better.
Conclusion
Arrays are like movie theater seats—great when you need quick access but not the best when you have to move things around a lot.
Key Takeaways:
- Fast Lookup: If you know the index, you get the item instantly. O(1)
- Slow Insertions/Deletions: Inserting in the middle shifts everything. O(n)
- Binary Search: If sorted, you can find items quickly. O(logn)
- Big O Matters! Choosing the right data structure depends on efficiency.
By understanding how arrays perform and where they struggle, you can choose the right tool for the job—just like picking the best seat in a packed movie theater! 🍿🎥