Navigating Linked Lists: Sequential Flexibility Explained

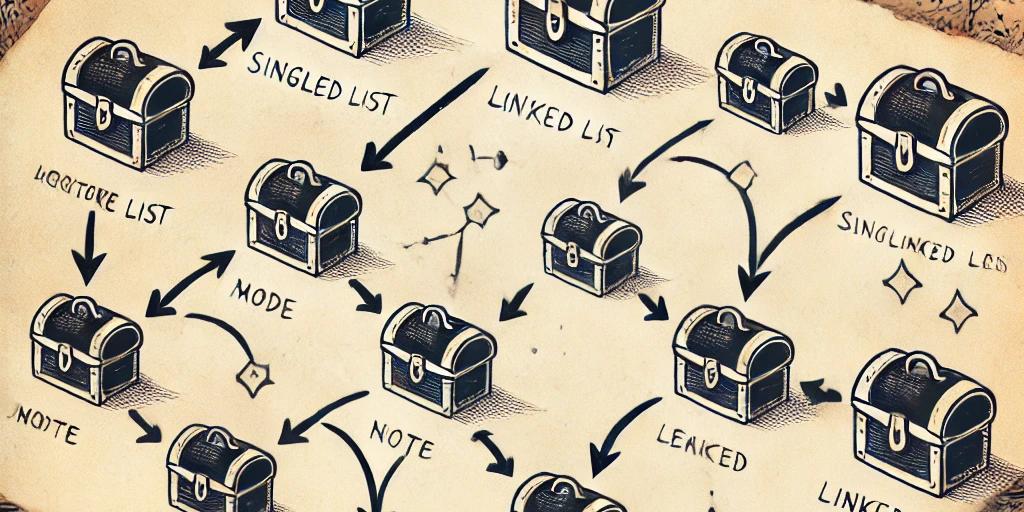

Understanding Data Structures Through Big O Notation
In our previous discussion on Big O notation, we explored how it helps us measure the efficiency of algorithms. Now, let’s take the next logical step: understanding data structures in the context of Big O.
Why Does Big O Matter for Data Structures?
Every data structure is built for specific tasks. Some are great at fast lookups, while others make insertions and deletions easier. Understanding Big O notation helps us choose the right tool for the job.
What is a Linked List?
A linked list is a way to store data sequentially, but instead of using fixed positions like an array, each element (called a node) holds two things:
- The actual data (like a name or a number).
- A pointer to the next node in the sequence.
Unlike an array, where all elements sit next to each other in memory, linked lists are spread out and connected by links.
Real-World Analogy: A Scavenger Hunt
Imagine a scavenger hunt where each clue leads to the next location. You can't jump straight to the last clue like an array; you have to follow the path step by step.
- If you start at the first clue (head node), you keep following the instructions (pointers) to the next until you reach the end.
- This means finding a specific item can take time, but adding or removing clues is very easy because you just change where one clue points.
Why Use Linked Lists?
✅ Good for:
- Fast Insertions/Deletions → You can insert a new node without shifting everything. (Big O: for inserting at the head)
- Dynamic Size → Unlike arrays, you don’t need to know the size beforehand. (No wasted space)
❌ Not good for:
- Slow Searching → To find an item, you must go node by node. (Big O: )
- Extra Memory Usage → Each node has to store a pointer, using more memory than an array.
Time Complexity Overview
Operation | Big O Notation | Explanation |
---|---|---|
Access (indexing) | O(n) | Like searching for a clue in a long list. |
Search | O(n) | You must check each clue one by one. |
Insert (at head) | O(1) | Adding a new clue at the start. |
Insert (middle/end) | O(n) | You have to read every clue until you reach the right one |
Delete (at head) | O(1) | Just remove the first clue |
Delete (middle/end) | O(n) | You have to read each clue before you can remove the one you want |
Example: Inserting a Node at the Head
Since linked lists don’t have fixed positions, inserting a new element at the beginning is super fast.
Linked List Insertion Code Example (TypeScript)
1class Node {
2 value: number;
3 next: Node | null;
4
5 constructor(value: number) {
6 this.value = value;
7 this.next = null;
8 }
9}
10
11class LinkedList {
12 head: Node | null = null;
13
14 insertAtHead(value: number): void {
15 const newNode = new Node(value);
16 newNode.next = this.head;
17 this.head = newNode;
18 }
19
20 printList(): void {
21 let current = this.head;
22 while (current) {
23 console.log(current.value);
24 current = current.next;
25 }
26 }
27}
28
29// Usage Example:
30const myList = new LinkedList();
31myList.insertAtHead(10);
32myList.insertAtHead(20);
33myList.insertAtHead(30);
34myList.printList(); // Output: 30, 20, 10
How It Works:
- We create a new node.
- The new node’s
next
pointer points to the old head. - We update the head to the new node.
🔹 Big O:O(1) because we don’t shift existing elements.
Common Mistakes (Pitfalls)
🚨 Mistake #1: Forgetting to Update Pointers
- If you remove a node but don’t update the previous node’s pointer, you might "lose" part of your list.
🚨 Mistake #2: Searching is Slow
- Unlike an array, you cannot jump to index 5 instantly. You must follow the pointers step by step.
🚨 Mistake #3: Memory Overhead
- Since each node stores extra pointers, linked lists take up more memory than arrays.
Best Practices
✔ Use Linked Lists When You Need Frequent Insertions/Deletions
✔ Consider Other Structures for Fast Lookups (like hash tables)
✔ Watch for Null Pointers – Always check if a node exists before accessing .next
Conclusion
🎯 Key Takeaways:
- Linked lists store elements with pointers instead of fixed positions.
- Inserting at the head is fast, but searching takes time .
- Best for flexible-sized data where quick insertions/deletions matter.
- Big O helps us understand when linked lists outperform arrays.
If you understand linked lists, you’re one step closer to mastering stacks, queues, and more advanced structures! 🚀